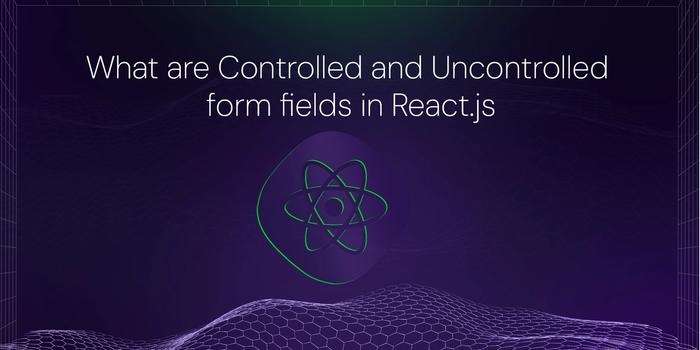
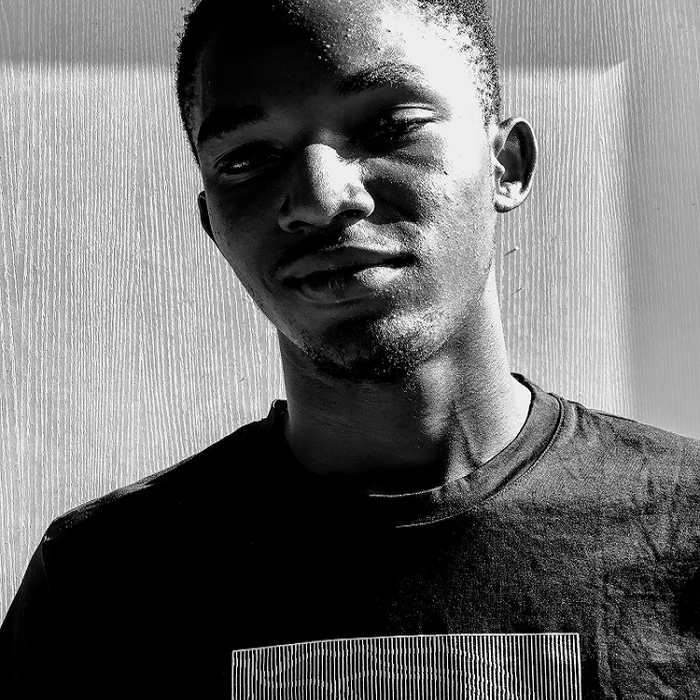
Henry Imoh
6 min readApr 08 2022
What are Controlled and Uncontrolled form fields in React.js
Form elements in HTML, such as <input/>
, <textarea>
, <select>
, and so on, often keep their inherent state and update it based on user input. We utilize state in every React component to make React apps interactive. The state of a component is initialized with a value and changed at some point in time based on user interactions with the application.
In React forms, input values are of two kinds, depending on your preference: uncontrolled and controlled inputs. To help you grasp the difference, we will go through both approaches to handling forms in React. The HTML technique utilizes "uncontrolled form fields," and the best practice, which uses "controlled form fields.”
Controlled Inputs
In controlled inputs, modifications and changes always occur in the form field. This is seen in the value prop passed to the component. Every character typed, even something as simple as a backspace, counts as a change. A significant part of a controlled component is that the state variable is the single source of truth.
We will utilize function components in this piece and React hooks. If you are not familiar with these concepts, do get acquainted with them.
Hooks, in a nutshell, are a cleaner, more succinct way of writing components. We will utilize a React Hook called useState()
to build a function component. This hook exposes a state variable and a function to update the variable. Let’s have a code example:
import React, { useState } from "react"; function App (props) { const [message, updateMessage] = useState(""); return ( <div className="App" style={{ height: "20px" }}> <div className="container"> <input type="text" placeholder="Enter message here.." value={message} onChange={(event) => updateMessage(event.target.value)} /> <p>the message is: {message}</p> </div> </div> ); }; export default App;
Here's a basic function component that renders a single input field on the page and reflects anything the user enters.
In the above function component called App
, we have a state named message
, and it can be updated using the updateMessage
method. The input element returned in the component updates the state variable using its onChange
event handler
A parent form
element is not required for an input field to be classified as controlled.
It might be challenging to work with controlled components. If the page contains a large number of input elements, each one must be given a value property and an event handler.
View the full demo here
Uncontrolled Inputs
There is no updating or altering of states when the input values are uncontrolled. Uncontrolled components keep track of their internal state, which means the component remembers what you entered into the field. That value can be manipulated by utilizing the ref keyword to fetch it whenever it is needed. The value you submit is the value you get with uncontrolled inputs.
Refs allow you to access DOM nodes or React elements formed during the render process. More on refs
Uncontrolled components behave more like HTML form elements. The DOM, not the component, stores the data for each input element.
Let’s see an example below:
import { useRef } from "react"; const NameForm = () => { const inputRef = useRef(null); const handleSubmit = () => { alert(inputRef.current.value); }; return ( <div> <input type="text" placeholder="Type..." ref={inputRef} /> <button onClick={handleSubmit}>Submit</button> </div> ); }; export default NameForm;
In the above example, we have created a ref
called inputRef
and attached it to the <input>
element using ref
attribute. Its value is displayed on the alert box upon clicking the submit button. View the full demo here
The next challenge might be when to use the controlled component and when to apply the uncontrolled component.
Working with controlled components on pages with numerous input fields might be problematic. An uncontrolled component is recommended in this scenario. Controlled components are appropriate when handling form validations, as you can validate the input values on each keystroke.
Conclusion
Both controlled and uncontrolled form fields have their own set of advantages. Depending on the scenario, you may need to use both or one of them. If you're constructing a basic form with little to no UI feedback and no need for immediate validation (that is, you will only confirm or need the values after you submit), you should use uncontrolled inputs with refs. The source of truth would remain in the DOM, making it faster and requiring less code.
Controlled input is the way to go if you need more UI feedback and want to account for every minor change in a specific input field.
About the author
I'm a front-end web developer and Content writer. I possess 2+ years of professional experience shipping high-end web products and writing technical content. I am passionate about photography, creating impactful content, and web products. I also possess design, technical writing, psychology, and data visualization skills.
More articles
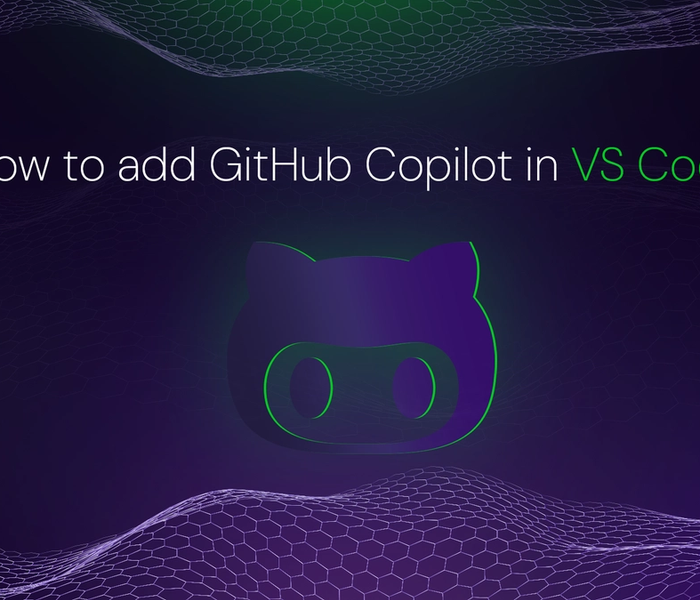
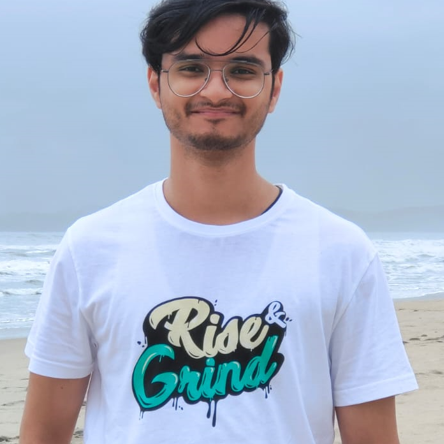
Akshat Virmani
6 min readAug 24 2024
How to add GitHub Copilot in VS Code
Learn how to add GitHub Copilot to Visual Studio Code for AI-assisted coding. Boost productivity, reduce errors, and get intelligent code suggestions in seconds.
Read Blog
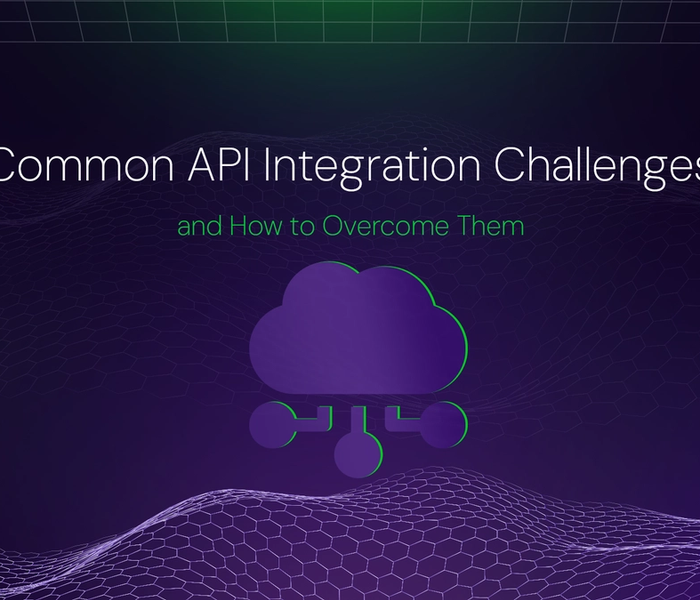
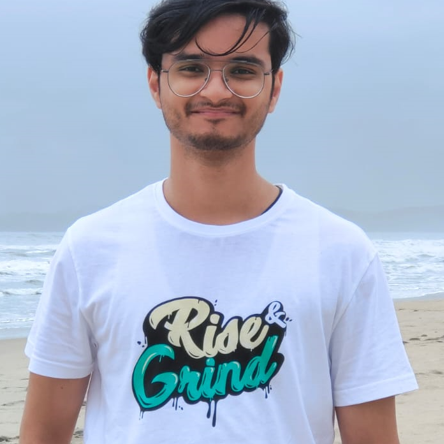
Akshat Virmani
6 min readAug 09 2024
Common API Integration Challenges and How to Overcome Them
Discover common API integration challenges and practical solutions. Learn how to optimize testing, debugging, and security to streamline your API processes efficiently.
Read Blog
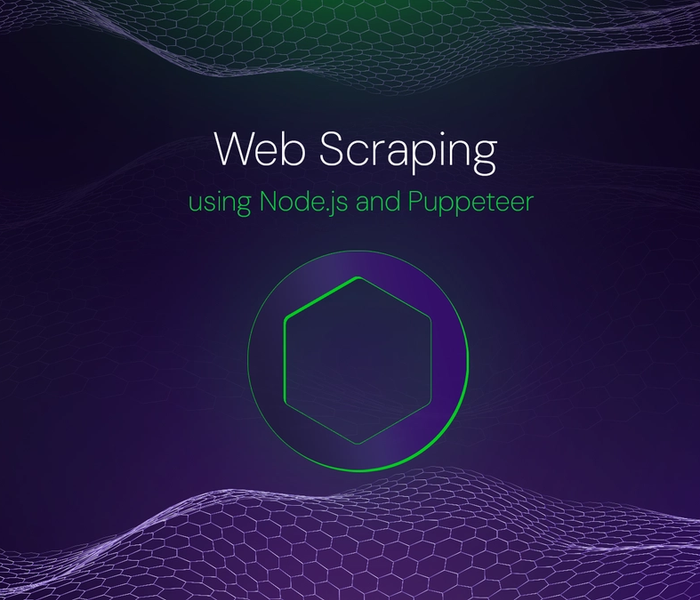
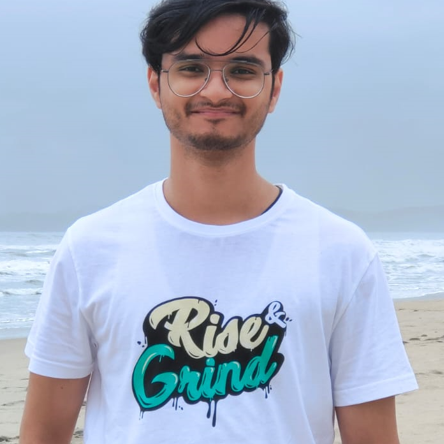
Akshat Virmani
6 min readJun 20 2024
Web Scraping using Node.js and Puppeteer
Step-by-step tutorial on using Node.js and Puppeteer to scrape web data, including setup, code examples, and best practices.
Read Blog